A WEB Server on the ESP32
A "Hello World!" WEB server
Writing a WEB server from scratch is not a trivial task, but then the need of a WEB server is so common that you would expect that some kind soul has done the job for you. On MicroPython you find several WEB servers ready for deployment. I have selected
picoweb
because it is small enough to be easily studied and it has all the functionality needed for the projects in this course. On the other hand picoweb depends on several modules that are not installed in MicroPython by default. I therefore collected everything that is needed and compiled picoweb into the MicroPython binary making it available for immediate use.
Implementing the Hello World! WEB server
As examples we provide 3 different versions of the Hello World WEB server:
- Version1 does not use picoweb at all but opens a socket and listens to requests. Once it sees a request it sends some HTML text, stored in the MicroPython source code
- Version 2 exports the HTML code to a separate HTML file. It uses picoweb and answers if either serverHost/ or serverHost/index.html is requested. The HTML file is read and its text returned to the caller
- Version 3 works in a similar fashion to Version 2 but the HTML file is gzipped. Since the ESP32 has little free "disk space", well, space in its flash, it is interesting to compress the HTML file to save resources.
Version 1
Version 1 is the basic WEB server we implemented in
BasicWEBServer
Version 2:
In the second version we will separate the HTML text from the server and store it in the html directory (to be created with ampy:
ampy mkdir /html) on the ESP32. Now we can use a ready made Web server for MicroPython,
picoweb
to serve the page.
picoweb and
utemplates (see exercise 2) are already included in the MicroPython binary.
Studying
example_webapp.py in the base directory of
picoweb will show you how to do this.
Since from now on we will have to upload several file to the ESP32 I provide shell scripts named
setup.sh which create directories like /html to store html files, /templates to store templates (see later) and /static to store static images. In fact it the script will check if the directories already exist on the ESP32 and create them only if not. Once the directories are created it stores the necessary html files, templates or images into their corresponding directory.
Version 3:
If we do not use an external SD card, then space for the HTML pages is rather limited. It may therefore be interesting to use gzip compresses pages. Modify your picoweb server to use gzipped HTML.
and finally a screen dump of the WEB pages as seen by the browser
You can download the code here:
https://iotworkshop.africa/pub/IoT_Course_English/WEBServerPicoweb/helloWorldWebServer.tar.gz
To make the programs run, first execute setup.sh. This will create the directories needed on the ESP32 file system and upload the html files into their correct places. Please have a look into this shell script to understand what it is doing. Then run the WEB server in thonny:
thonny helloWorldWebServerV2.py
The last line printed tells you that you can access the server on the URL: 192.168.0.46 with your browser.
Adding Temperature and Humidity Measurements
From the Hello World Web server to a server that actually shows measurements is only a small step. In the following example we create a table on HTML into which we insert the measurements taken with the SHT30 (see
SHT30NopI2CTemperatureAndHumiditySensor) This is done with templates using the
utemplate module in MicroPython. Have a look at the picoweb examples to see how this can be done.
https://iotworkshop.africa/pub/IoT_Course_English/WEBServerPicoweb/sht30.tar.gz
The tar archive contains:
- sht30Server.py: The WEB server, based on picoweb, that must be run on the ESP32.
- templates/sensor.tpl: The template file that must be stored in the templates directory of the ESP32
- setup.sh: a shell script that creates the directories needed on the ESP32 (if they do not exists already) and uploads the template file into its correct place
- timeStamp.py: a test program creating a time stamp from the current time (not needed here)
Making the WEB page interactive
The WEB server above has one major flaw: for each measurement the complete page must be reloaded. Wouldn't it be nice if we could keep the table and only replace the measurement results and time stamp?
Such types of actions can be accomplished with javascript, a full programming language for WEB programming running on the client side, in the browser. Teaching also javascript would largely exceed the scope of this course. However, if you don't know javascript well enough (as was the case for me!) here is a nice tutorial which will put you on track quickly:
https://www.w3schools.com/js/DEFAULT.asp
Controlling the ESP32 User Led
Before looking at how to display the SHT30 measurements on our WEB server, let's first try to give WEB control to the user LED on the ESP32 CPU board. We want to create a WEB page allowing to switch this LED on and off. The icons for the LED are attached to this page:
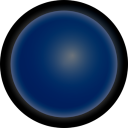
and
We will start with the light bulb example from the
introduction
in the javascript tutorial, replacing the light bulb with a blue LED (simulating the LED on the ESP32 CPU board.
Whenever you click the "Turn on the light" button the icon switches to led-blue-on-128.png showing a lighted blue LED while the other button shows the LED turned off. Just select the
dummyLED.html in the tar archive with the browser and try.
https://iotworkshop.africa/pub/IoT_Course_English/WEBServerPicoweb/ledControl.tar.gz
Communication between the WEB client, on which javascript is running, and the WEB server on the ESP 32 can be accomplished with
Asynchronous
JavaScript
And
XML (AJAX). To understand this you will have to go through yet another tutorial:
the AJAX tutorial
.
Essentially you must create aXMLHttpRequest, which you send from the client to the server and to which the server will respond. You use the answer from the server to update the HTML page using javascript. The
ledControl.html file defines 2 functions:
ledOn() and
ledOff() sending an XMLHttpRequest to the WEB server. The WEB server switches the user LED on or off and answers with the current state of the LED. This information is used be the javascript code in the html file to show a lighted or dark LED icon.
ajaxLEDServer.py in the tar archive is the WEB server to be run on the ESP32, /html/ledControl.html is the HTML and javascript file and setup.sh is a shell script uploading all the necessary files to their correct places in the ESP32 file system.
Making Temperature and Humidity measurements available to the WEB page through AJAX
With the knowledge on how to measure temperature and humidity and the knowledge on how to communicate between the WEB client and the WEB server using AJAX we have all we need to create a WEB page showing periodic temperature and humidity measurements. For measurement of other parameters we only need to implement the driver code reading out the sensor in addition.
Plotting the result on the client side is another story. However, a very powerful javascript library is available to do exactly this:
https://www.highcharts.com/docs/index
.
Explaining this library would again largely exceed the scope of this course. Have a look at the documentation and in particular the examples given with it to understand how you can plot measurement data taken over a long period. An example for the SHT30 is given here:
https://iotworkshop.africa/pub/IoT_Course_English/WEBServerPicoweb/highCharts.tar.gz.
The plot shows temperature and humidity data taken at intervals of 30s during a whole night. It allows to
- zoom into a particular time period
- save the acquired data on a .csv file for offline evaluation
- and much more.
--
Uli Raich - 2020-04-28
Comments